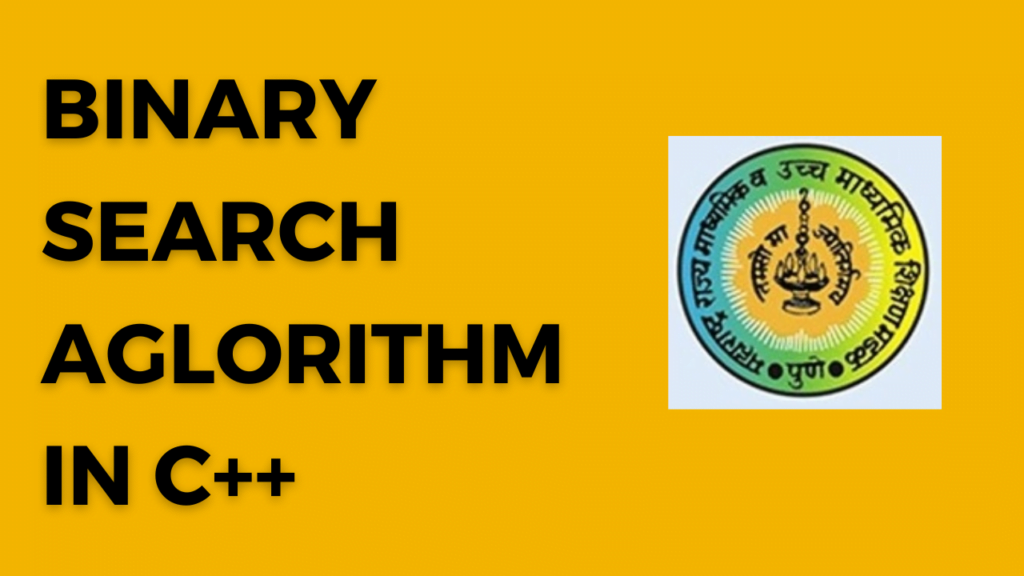
write a program in C++ that first initialize an array of given 10 sorted real numbers. The program must verify whether the given element is to an array or not, using a binary search technique.
The element is to be entered at the time of execution. If the number is found, the program should print its position in the array otherwise it should print the number that is not found.
what is Binary Search Technique?
Binary Search is a searching algorithm used in a sorted array by repeatedly dividing the search interval in half. The idea of binary search is to use the information that the array is sorted and reduce the time complexity.
Basic steps to perform binary search technique
- Sort the array in ascending order.
- Set the low index to the first element of the array and the high index to the last element.
- Set the middle index to the average of the low and high indices.
- If the element at the middle index is the target element, return the middle index.
- If the target element is less than the element at the middle index, set the high index to the middle index – 1.
- If the target element is greater than the element at the middle index, set the low index to the middle index + 1.
- Repeat steps 3-6 until the element is found or it is clear that the element is not present in the array.
Let’s quickly go through the program Binary search Technique
#include<iostream.h>
#include<conio.h>
#include<stdlib.h>
void main()
{
float a[10], p;
int i, top, bot, mid;
cout << "Type the number in ascending order" << "\n";
for (i = 0;i < 10;i++) { cin >> a[i]; }
top = 0; bot = 9;
cout << "Type the number you want to search \n";
cin >> p;
mid = (top + bot) / 2;
while ((top <= bot) && (a[mid] != p))
{
if (p < a[mid])
bot = mid - 1;
else
top = mid + 1;
mid = (top + bot) / 2;
}
if (a[mid] == p)
{
cout << "the number is at position" << (mid + 1) << "\n";
}
else
cout << "The number is not found\n";
getch();
}